The Power of 10: Implement Nasa Rules to Your Roblox Studio Development Workflow
Unlock the secrets to better coding in Roblox Studio with insights inspired by NASA's 10 programming rules.
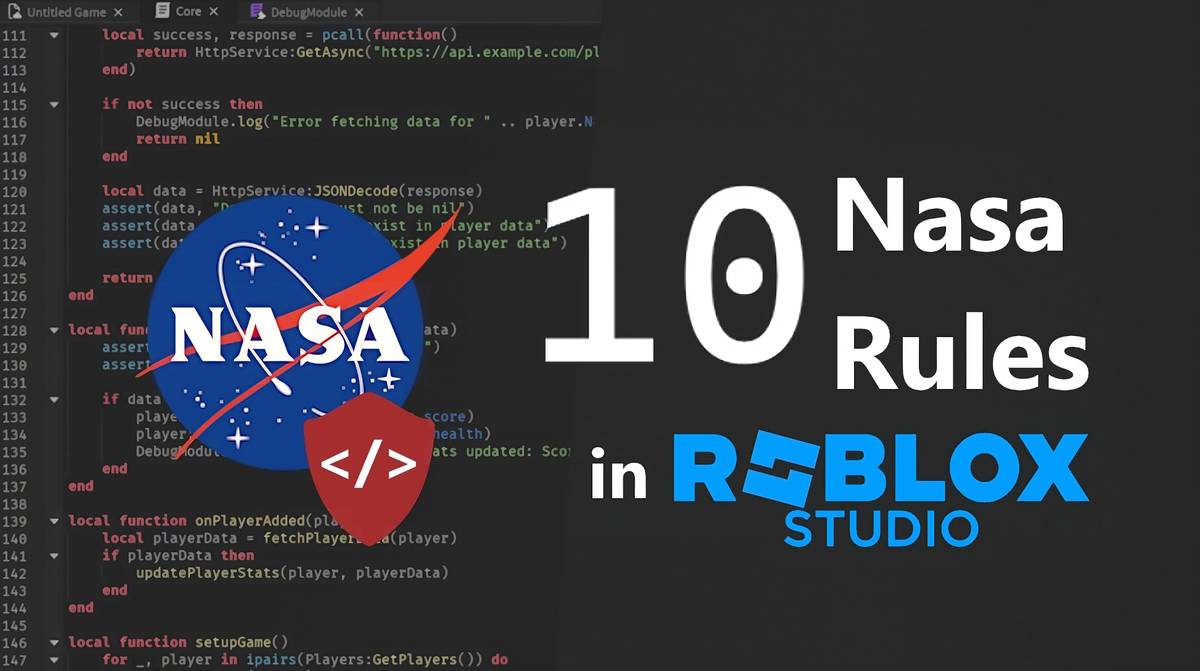
Introduction
In Roblox Studio, writing a clean, efficient, and maintainable code is crucial. NASA follows 10 programming rules they have developed, originally designed for high-stakes aerospace software, that can be adapted to enhance your coding practices in Roblox Studio using Luau scripting language. In this 10 steps long blog tutorial, you will find out how you can implement these rules in your Roblox projects, along with alternatives where necessary.
1. Avoid Complex Flow Constructs
Avoid looping functions at all cost unless there is no other alternative, as they can lead to stack overflow errors and make your code harder to manage. Instead, stick to simple control structures like for, while, and repeat for looping, which provide clearer logic and better performance in your scripts.
--Limit this:
function SpawnNpc(plr: Player)
local Npc = game.ReplicatedStorage.NPC:Clone()
Npc.Parent = workspace
task.wait(1) --> correct way to use "wait" as it's not deprecated
plr.leaderstats.EnemyCount.Value += 1
SpawnNpc(plr) -->This run the function again
end
SpawnNpc(game.Players:GetPlayers()[1])
--Use this:
while true do
local Npc = game.ReplicatedStorage.NPC:Clone()
Npc.Parent = workspace
task.wait(1) --> correct way to use "wait" as it's not deprecated
plr.leaderstats.EnemyCount.Value += 1
end
2. Set a Loop Limit
When writing loops in Roblox Luau, it's important to ensure that they have defined boundaries. This means specifying the exact number of iterations or providing a clear condition for when the loop should stop. Loops with fixed bounds are easier to debug, and optimize compared to loops with non-limited runs.
You can achieve this by using built-in for and repeat until loops, which have defined limits by default. Occasionally, you can also use a while loop combined with a break statement to stop the loop when a certain condition is met. This approach ensures that your code remains predictable and manageable.
--> This will run 10 times.
for i = 1, 10 do
print(i)
end
--> This will run continuously for 2 seconds.
local CurrentTime = os.clock()
local cooldown = 2
repeat
print(CurrentTime)
task.wait()
until os.clock() - CurrentTime >= cooldown
--> This will run continuously until the random number is 1.
local
while true do
local randomNumber = math.random(1,10)
if randomNumber == 1 then
break
end
task.wait()
end
In some cases, an endless loop may be necessary for certain logic, such as an NPC checking for players, as shown below. Notice that there is still a break statement within an if condition, meaning the loop can end. However, this will only happen when the NPC's health reaches zero. Without the break, the loop could lead to some issues, causing errors and affecting optimization.
Since the code is placed under the NPC model, it will reset and function correctly when the NPC respawns. With that in mind, you can also place the script in ServerScriptService and use functions that respond to NPC death events to manage the workflow more effectively. This approach allows for a more structured and efficient handling of NPC behavior without relying solely on endless loops.
while true do
--> Check if the NPC's health is greater than 0
if humanoid.Health <= 0 then
print("NPC is dead. Stopping movement.")
break -- Exit the loop if the NPC's health is 0
end
if targetPlayer and targetPlayer.Character then
local targetPosition = targetPlayer.Character.HumanoidRootPart.Position
--> Create a path to the target position
local path = PathfindingService:CreatePath({
AgentRadius = 2,
AgentHeight = 5,
AgentCanJump = true,
AgentJumpHeight = 10,
AgentMaxSlope = 45,
})
path:ComputeAsync(NPC.Position, targetPosition)
path:MoveTo(NPC)
--> Wait for the path to finish moving
path:MoveToFinished:Wait()
else
print("Target player not found.")
end
task.wait()
end
end
3. Avoid Creating New Memory During the Run
Instead of dynamically creating objects during gameplay, predefine your objects and reuse them. For example, use existing local variables to manage frequently used items, which can improve performance and reduce memory usage.
--> Predefined variables
local _workspace = workspace
local CoinPos = Vector.new(2,5,2)
--> Code ex
local function SpawnCoin(coin)
coin.Position = CoinPos --> CoinPos variable is reused
coin.Parent = _workspace --> _workspace variable is reused
SpawnCoin(_workspace.Coin:Clone())
To avoid dynamic memory allocation, create a pool of objects that you can reuse throughout your game. When an object is no longer needed, return it to the pool instead of destroying it. This reduces the overhead of constantly creating and destroying objects.
local objectPool = {} -- Initializes an empty table to act as the object pool
-- Function to get an object from the pool or create a new one if the pool is empty
function getObject()
if #objectPool > 0 then
return table.remove(objectPool) -- Returns an object from the pool if available
else
return game.ReplicatedStorage.ObjectTemplate:Clone() -- Creates a new object if the pool is empty
end
end
-- Function to release an object back into the pool
function releaseObject(obj)
obj:SetPrimaryPartCFrame(CFrame.new()) -- Resets the object's position
table.insert(objectPool, obj) -- Inserts the object back into the pool
end
4. Shorten Functions
It is advisable to keep your functions concise. As a general rule, functions should be limited to a few lines of code. If a function is getting too long, consider breaking it into smaller helper functions. This improves readability and makes debugging way easier as you can identify where the issue located.
local function createLeaderstats(player)
local leaderstats = Instance.new("Folder")
leaderstats.Name = "leaderstats"
leaderstats.Parent = player
return leaderstats
end
local function createScore(leaderstats)
local score = Instance.new("IntValue")
score.Name = "Score"
score.Value = 0
score.Parent = leaderstats
end
local function createHealth(leaderstats)
local health = Instance.new("IntValue")
health.Name = "Health"
health.Value = 100
health.Parent = leaderstats
end
local function handlePlayerJoin(player)
local leaderstats = createLeaderstats(player)
createScore(leaderstats)
createHealth(leaderstats)
print(player.Name .. " has joined the game with 100 health.")
end